8. Gestion de l'accès à l'application▲
8-A. Changement de la page d'accueil▲
On va changer la page d'accueil et on mettra authentification.jsp comme page d'accueil.
On ouvre le fichier web.xml puis on clique sur l'onglet pages
On clique sur browse puis on choisit Authentification.jsp
8-B. Création du Bean «AuthenticationBean »▲
La dernière étape de notre projet sera la création du bean pour l'authentification et de faire la redirection entre la page d'authentification et les autres pages.
On commence par la création des deux interfaces DAO et Service pour ce bean.
package
Interfaces;
/**
*
@author
Jihed
*/
import
Entity.Personnel;
public
interface
AuthenticationService {
public
Personnel findByLoginAndPassword
(
String login, String pass);
}
package
Interfaces;
import
Entity.Personnel;
/**
*
@author
Jihed
*/
public
interface
AuthenticationDAO {
public
Personnel findByLoginAndPassword
(
String login, String pass);
}
Puis on passe à l'implémentation
package
Implementation.dao;
import
Entity.Personnel;
import
Interfaces.AuthenticationDAO;
import
org.springframework.orm.hibernate3.support.HibernateDaoSupport;
/**
*
@author
Jihed
*/
public
class
AuthenticationDaoImpl extends
HibernateDaoSupport implements
AuthenticationDAO {
public
Personnel findByLoginAndPassword
(
String login, String pass) {
try
{
Personnel pers =
(
Personnel) getHibernateTemplate
(
).find
(
" from Personnel pers where pers.persLogin ='"
+
login +
"' and pers.persPassword ='"
+
pass +
"'"
).get
(
0
);
return
pers;
}
catch
(
Exception re) {
re.printStackTrace
(
);
return
null
;
}
}
}
package
Implementation.service;
import
Entity.Personnel;
import
Interfaces.AuthenticationDAO;
import
Interfaces.AuthenticationService;
public
class
AuthenticationServiceImpl implements
AuthenticationService {
private
AuthenticationDAO loginDao;
public
Personnel findByLoginAndPassword
(
String login, String pass) {
return
loginDao.findByLoginAndPassword
(
login, pass);
}
public
AuthenticationDAO getLoginDao
(
) {
return
loginDao;
}
public
void
setLoginDao
(
AuthenticationDAO loginDao) {
this
.loginDao =
loginDao;
}
}
Déclaration dans le fichier de configuration de Spring
<bean
id
=
"loginDao"
class
=
"Implementation.dao.AuthenticationDaoImpl"
>
<property
name
=
"hibernateTemplate"
ref
=
"hibernateTemplate"
/>
</bean>
<bean
id
=
"loginService"
class
=
"Implementation.service.AuthenticationServiceImpl"
>
<property
name
=
"loginDao"
ref
=
"loginDao"
/>
</bean>
Création d'un nouveau bean qu'on appelle « AuthenticationBean »
package
Beans;
import
Entity.Personnel;
import
Interfaces.AuthenticationService;
import
java.text.SimpleDateFormat;
import
java.util.Date;
import
javax.faces.context.ExternalContext;
import
javax.faces.context.FacesContext;
/**
*
@author
Hsan
*/
public
class
AuthenticationBean extends
messageBean {
private
AuthenticationService loginService;
private
String login;
private
String password;
private
String today;
private
Personnel pers;
private
String message;
public
AuthenticationBean
(
) {
}
public
String getLogin
(
) {
return
login;
}
public
void
setLogin
(
String login) {
this
.login =
login;
}
public
String getPassword
(
) {
return
password;
}
public
void
setPassword
(
String password) {
this
.password =
password;
}
public
String getToday
(
) throws
Exception {
Date maDateAvecFormat =
new
Date
(
);
SimpleDateFormat dateStandard =
new
SimpleDateFormat
(
"EEEE dd MMMM yyyy"
);
today =
""
+
dateStandard.format
(
maDateAvecFormat);
dateStandard =
null
;
return
today;
}
public
void
setToday
(
String td) {
this
.today =
td;
}
public
String connecter
(
) {
String droit =
null
;
message =
""
;
try
{
pers =
loginService.findByLoginAndPassword
(
login, password);
if
(
pers !=
null
) {
if
(
pers.getPersDroit
(
).equals
(
"user"
)) {
droit =
"user"
;
}
else
if
(
pers.getPersDroit
(
).equals
(
"admin"
)) {
droit =
"admin"
;
}
System.out.println
(
"********DROIT*****"
+
droit);
return
droit;
}
else
{
message =
"Échec de connexion, vérifiez votre login et mot de passe !"
;
style_message =
"err_message"
;
this
.login =
""
;
this
.password =
""
;
return
"invalide"
;
}
}
catch
(
Exception fe) {
fe.printStackTrace
(
);
message =
"Échec de connexion, vérifiez votre login et mot de passe !"
;
this
.login =
""
;
this
.password =
""
;
style_message =
"err_message"
;
return
"invalide"
;
}
}
public
String deconnecter
(
) {
try
{
ExternalContext ExtContext =
FacesContext.getCurrentInstance
(
).getExternalContext
(
);
ExtContext.getSessionMap
(
).clear
(
);
}
catch
(
Exception ex) {
ex.printStackTrace
(
);
}
return
"ok"
;
}
public
String getMessage
(
) {
return
message;
}
public
void
setMessage
(
String message) {
this
.message =
message;
}
public
AuthenticationService getLoginService
(
) {
return
loginService;
}
public
void
setLoginService
(
AuthenticationService loginService) {
this
.loginService =
loginService;
}
public
Personnel getPers
(
) {
return
pers;
}
public
void
setPers
(
Personnel pers) {
this
.pers =
pers;
}
}
8-C. Liaison avec la page Authentification▲
<%--
Document : Authentification
Created on : 25 janv. 2010, 21:30:57
Author : jihed
--%>
<%@taglib
uri
=
"http://richfaces.org/a4j"
prefix
=
"a4j"
%>
<%@taglib
uri
=
"http://richfaces.org/rich"
prefix
=
"rich"
%>
<%@taglib
prefix
=
"f"
uri
=
"http://java.sun.com/jsf/core"
%>
<%@taglib
prefix
=
"h"
uri
=
"http://java.sun.com/jsf/html"
%>
<%@page
contentType
=
"text/html"
pageEncoding=
"UTF-8"
%>
<!
DOCTYPE HTML PUBLIC
"-//W3C//DTD HTML 4.01 Transitional//EN"
"http://www.w3.org/TR/html4/loose.dtd"
>
<f
:
view
>
<h
:
form
>
<html>
<head>
<meta http-equiv=
"Content-Type"
content=
"text/html; charset=UTF-8"
>
<title>
Authentification</title>
</head>
<body >
<rich
:
spacer
height=
"200px"
></rich
:
spacer
>
<center >
<rich
:
panel
id=
"logpan"
style=
"background-image:url(#{facesContext.externalContext.requestContextPath}/images/ajax/userauth.png);
background-repeat:no-repeat;background-position:-35px -15px;
;width:400px;"
header=
"Authentification"
styleClass=
"panel_3"
>
<h
:
panelGrid
columns=
"3"
>
<h
:
outputText
value=
"Login:"
/>
<h
:
inputText
id=
"log"
value=
"#{LoginBean.login}"
required=
"true"
requiredMessage=
"champs obligatoire"
/>
<rich
:
message
for=
"log"
style=
"color: red"
/>
<h
:
outputText
value=
"Mot de passe :"
/>
<h
:
inputSecret
id=
"mdp"
value=
"#{LoginBean.password}"
required=
"true"
requiredMessage=
"champs obligatoire"
/>
<rich
:
message
for=
"mdp"
style=
"color: red"
/>
</h
:
panelGrid
>
<rich
:
spacer
height=
"30px"
></rich
:
spacer
>
<a4j
:
commandButton
value =
"Connexion"
action=
"#{LoginBean.connecter}"
reRender=
"logpan"
/>
</rich
:
panel
>
</center>
</body>
</html>
</h
:
form
>
</f
:
view
>
8-D. Ajout des « navigation rules »▲
Les « navigation rules » permettent de définir les redirections entre les pages web dans notre application. Dans notre exemple si le Bean « authentification » va retourner la valeur « invalid » on va rester sur la même page « Authentification ».
Si le Bean retourne la valeur « user » on va être redirigé vers la page « Personnel » et si le bean retourne la valeur « admin » on va être redirigé vers la page « Interventions ».
Ajout des navigations rules
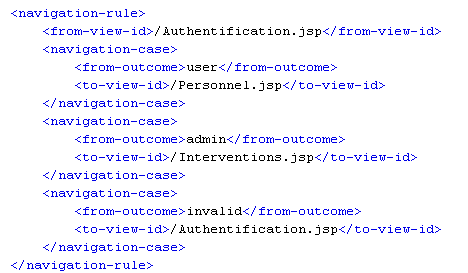
8-E. Changement de la page Entête▲
On va changer un peu dans la structure de l'entête pour pouvoir afficher les détails de la personne connectée.
<%--
Document : Entete
Created on : 25 janv. 2010, 21:47:07
Author : jihed
--%>
<%@taglib
uri
=
"http://richfaces.org/a4j"
prefix
=
"a4j"
%>
<%@taglib
uri
=
"http://richfaces.org/rich"
prefix
=
"rich"
%>
<%@taglib
prefix
=
"f"
uri
=
"http://java.sun.com/jsf/core"
%>
<%@taglib
prefix
=
"h"
uri
=
"http://java.sun.com/jsf/html"
%>
<%@page
contentType
=
"text/html"
pageEncoding=
"UTF-8"
%>
<!
DOCTYPE HTML PUBLIC
"-//W3C//DTD HTML 4.01 Transitional//EN"
"http://www.w3.org/TR/html4/loose.dtd"
>
<h
:
panelGrid
columns=
"12"
>
<rich
:
spacer
width=
"50px"
/>
<h
:
outputText
id=
"dtjr"
value=
"#{LoginBean.today}"
styleClass=
"titre_gris"
/>
<rich
:
spacer
width=
"225px"
/>
<h
:
outputText
id=
"user"
value=
"#{LoginBean.pers.persNom} #{LoginBean.pers.persPrenom}"
styleClass=
"titre_bleu"
/>
<rich
:
spacer
width=
"6px"
/>
<h
:
outputText
value=
" | "
styleClass=
"titre2"
/>
<rich
:
spacer
width=
"6px"
/>
<h
:
outputText
id=
"service"
value=
"#{LoginBean.pers.service.servLib}"
styleClass=
"titre_bleu"
/>
<rich
:
spacer
width=
"225px"
/>
<h
:
outputText
value=
"Club CFEM 2010"
styleClass=
"titre_gris"
/>
<rich
:
spacer
width=
"50px"
/>
<h
:
form
>
<h
:
panelGrid
columns=
"3"
>
<a4j
:
commandButton
image=
"/images/ajax/home.gif"
onclick=
"document.location.href='#{facesContext.externalContext.requestContextPath}/faces/Authentification.jsp'"
>
<rich
:
toolTip
showDelay=
"500"
>
Acceuil
</rich
:
toolTip
>
</a4j
:
commandButton
>
<rich
:
spacer
width=
"2px"
/>
<a4j
:
commandButton
action=
"#{LoginBean.deconnecter}"
id=
"dec_btn"
image=
"/images/ajax/lock.gif"
onclick=
"document.location.href='#{facesContext.externalContext.requestContextPath}/faces/Authentification.jsp'"
>
<rich
:
toolTip
showDelay=
"500"
>
Déconnexion
</rich
:
toolTip
>
</a4j
:
commandButton
>
</h
:
panelGrid
>
</h
:
form
>
</h
:
panelGrid
>